Get your ultimate Python Cheatsheet! Learn Python fast with our easy-to-use guide, packed with expert tips and tricks.
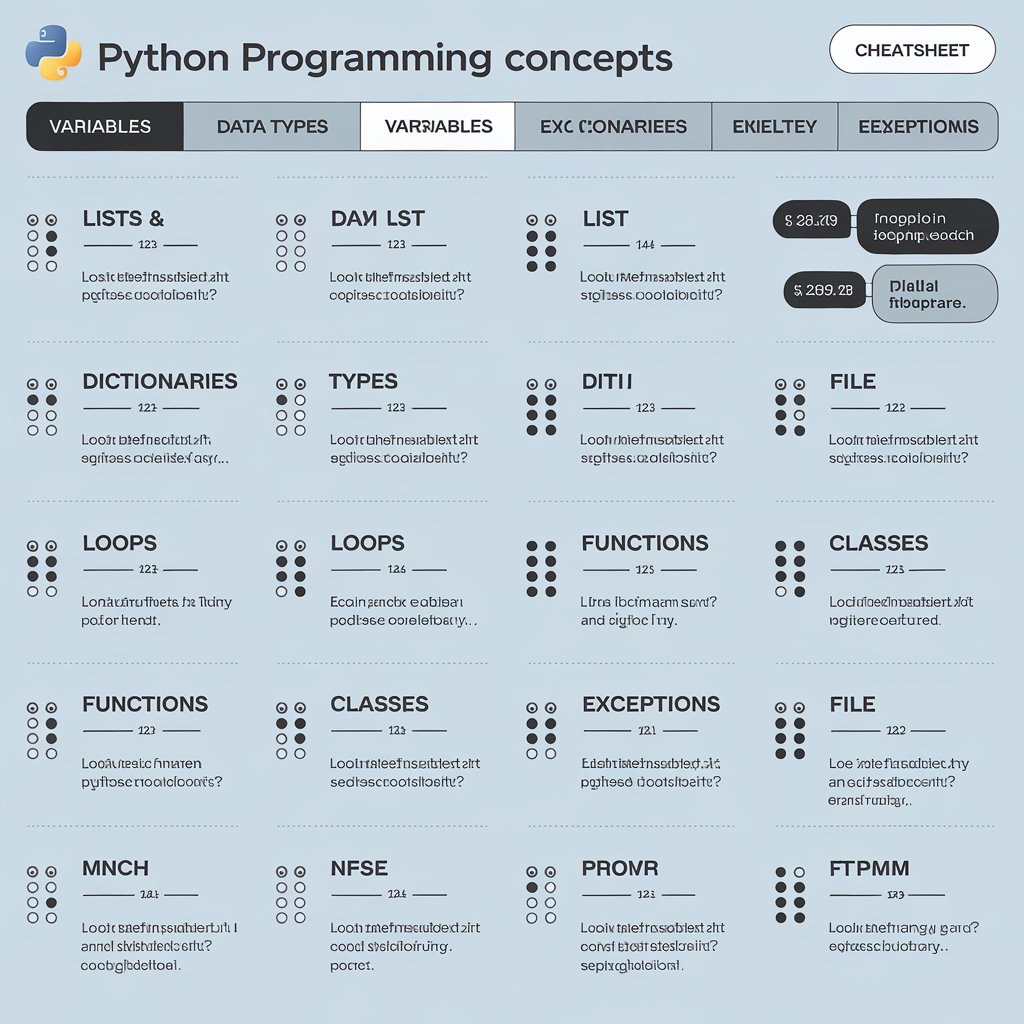
This Python cheatsheet serves as a concise reference for beginner and experienced programmers. If you are looking for a quick Python list cheatsheet or a comprehensive programming cheat sheet, this guide covers fundamental to advanced Python programming concepts.
Downloadable PDF: You can download a printable version of this python cheatsheet in PDF format.
1. Basic Syntax and Data Types
Python’s rules for writing code are simple and clear. Python uses spaces (indentation) at the beginning of lines to show where a code block starts and ends, while other programming languages use a lot of curly braces and semicolons.
Showing Output to the User
print("Content that you want to print on screen")
var1 = "Asad"
print("Hi, my name is:", var1)
Taking Input from the User
var1 = input("Enter your name: ")
print("My name is:", var1)
# Type casting for other data types
var2 = int(input("Enter an integer value: "))
print(var2)
var3 = float(input("Enter a float value: "))
print(var3)
Variables and Assignment
# Variable naming conventions
my_variable = 42 # Snake case recommended
camelCase = "Not preferred in Python"
CONSTANT_VALUE = 3.14159 # All uppercase for constants
# Multiple assignment
x, y, z = 1, 2, 3
# Type conversion
integer_value = int("123")
float_value = float(42)
string_value = str(3.14)
Type Checking
x = 42
print(type(x)) # <class 'int'>
isinstance(x, int) # True
Data Types
Python supports several core data types:
- Numeric Types
int
: Whole numbers (e.g., 10, -5, 0)float
: Decimal numbers (e.g., 3.14, -0.5)complex
: Complex numbers (e.g., 3+4j)
- Sequence Types
list
: Mutable, ordered collectiontuple
: Immutable, ordered collectionrange
: Sequence of numbers
- Text Type
str
: String, sequence of Unicode characters
- Mapping Type
dict
: Key-value pairs
- Set Types
set
: Unordered collection of unique elementsfrozenset
: Immutable version of set
Operators
Python provides a rich set of operators for manipulating data:
- Arithmetic Operators:
+
,-
,*
,/
,%
(modulo),**
(exponentiation),//
(floor division) - Comparison Operators:
==
(equal to),!=
(not equal to),>
,<
,>=
,<=
- Logical Operators:
and
,or
,not
- Assignment Operators:
=
,+=
,-=
,*=
,/=
,%=
,**=
,//=
- Bitwise Operators:
&
(AND),|
(OR),^
(XOR),~
(NOT),<<
(left shift),>>
(right shift) - Membership Operators:
in
,not in
- Identity Operators:
is
,is not
2. Control Flow
Control flow statements dictate the execution path of your program:
Conditional Statements
if condition1:
# Code to execute if condition1 is True
elif condition2:
# Code to execute if condition2 is True
else:
# Code to execute if all conditions are False
# Example
a = 15
b = 20
c = 12
if (a > b and a > c):
print(a, "is greatest")
elif (b > c and b > a):
print(b, " is greatest")
else:
print(c, "is greatest")
Loops
for loop: Iterates over a sequence (e.g., list, tuple, string).
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# Example with range() function
for i in range(1, 101, 1):
print(i)
range function: range(start, stop, step) generates a sequence of numbers.
# Display all even numbers between 1 to 100
for i in range(0, 101, 2):
print(i)
while loop: Repeats a block of code as long as a condition is True.
count = 0
while count < 5:
print(count)
count += 1
# Example
i = 1
while (i <= 100):
print(i)
i = i + 1
Loop Control Statements
break: Terminates the loop prematurely.
for i in range(1, 101, 1):
print(i, end=" ")
if (i == 50):
break
else:
print("Mississippi")
print("Thank you")
continue: Skips the current iteration and proceeds to the next.
for i in [2, 3, 4, 6, 8, 0]:
if (i % 2 != 0):
continue
print(i)
- pass: Acts as a placeholder, doing nothing.
- Indentation: Python uses indentation to define code blocks. Consistent indentation is crucial for proper execution.
3. Functions
Functions encapsulate reusable blocks of code, promoting modularity and organization
Defining a Function
def greet(name, greeting="Hello"):
"""Docstring describing function purpose"""
return f"{greeting}, {name}!"
# Default arguments
def power(base, exponent=2):
return base ** exponent
# Variable-length arguments
def sum_all(*args):
return sum(args)
# Keyword arguments
def user_profile(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
Return Values: Return
statement return a value from a function.
Arguments:
- Positional Arguments: Passed in the order they are defined.
- Keyword Arguments: Passed with the name of the parameter.
- Default Arguments: Provide a default value if no argument is passed.
- Variable-Length Arguments: Allow a function to accept an arbitrary number of arguments.
Lambda Functions
# Inline, anonymous functions
multiply = lambda x, y: x * y
print(multiply(3, 4)) # 12
4. Data Structures
Python has flexible data formats that help you organize and handle data easily.
Lists
Ordered mutable collections of items. Refer to the Python list cheatsheet section for a detailed overview of list manipulation.
my_list = [1, 2, 3, "apple", True]
my_list.append(4) # Add an item
my_list.remove("apple") # Remove an item
# List methods
my_list.index(2) # Returns the index of the first occurrence of 2
my_list.extend([5, 6]) # Extends the list with elements from another iterable
my_list.insert(1, "orange") # Inserts "orange" at index 1
my_list.pop(3) # Removes and returns the element at index 3
my_list.clear() # Removes all elements from the list
my_list.count(2) # Returns the number of times 2 appears in the list
my_list.reverse() # Reverses the order of the list
my_list.sort() # Sorts the list in ascending order
Tuples
Ordered, immutable collections of items.
my_tuple = (1, 2, 3, "apple", True)
# Tuple methods
my_tuple.count(2) # Returns the number of times 2 appears in the tuple
my_tuple.index("apple") # Returns the index of the first occurrence of "apple"
Dictionaries
Unordered collections of key-value pairs.
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
my_dict["age"] = 31 # Update a value
my_dict["country"] = "USA" # Add a key-value pair
# Dictionary functions and methods
len(my_dict) # Returns the number of key-value pairs
my_dict.clear() # Removes all items from the dictionary
my_dict.get("name") # Returns the value associated with the key "name"
my_dict.items() # Returns a view object with key-value pairs
my_dict.keys() # Returns a view object with the keys
my_dict.values() # Returns a view object with the values
my_dict.update({"country": "Canada"}) # Updates the dictionary with new key-value pairs
Sets
Unordered collections of unique items.
my_set = {1, 2, 3, 3, 4} # {1, 2, 3, 4}
my_set.add(5) # Add an item
my_set.remove(3) # Remove an item
# Set methods
my_set.discard(2) # Removes 2 from the set if it exists
my_set.intersection({3, 4, 5}) # Returns a new set with elements common to both sets
my_set.issubset({1, 2, 3, 4, 5}) # Returns True if my_set is a subset of the other set
5. Strings
Strings are sequences of characters and are immutable in Python:
- String Literals: Define strings using single (
'
), double ("
), or triple ('''
or"""
) quotes. - String Formatting:
- Old Style:
%
operator - New Style:
.format()
method - f-strings: Introduced in Python 3.6
- Old Style:
- String Methods: Python provides a plethora of built-in string methods for manipulation:
lower(), upper()
strip(), lstrip(), rstrip()
split(), join()
replace()
find(), index()
isalnum(), isalpha(), isdecimal(), isdigit(), islower(), isspace(), isupper()
- and many more…
Escape Sequences: Special characters used to represent certain formatting actions within strings.
print("\n") # Newline
print("\\") # Backslash
print("\'") # Single quote
print("\t") # Tab
print("\b") # Backspace
print("\ooo") # Octal value
print("\xhh") # Hex value
print("\r") # Carriage return
Indexing and Slicing: Access individual characters or substrings using indices.
my_string = "Hello, world!"
print(my_string[0]) # Output: H
print(my_string[1:5]) # Output: ello
6. File Handling
Interact with files to read and write data.
Reading and Writing
# Reading
with open('file.txt', 'r') as file:
content = file.read()
lines = file.readlines()
# Writing
with open('output.txt', 'w') as file:
file.write("Hello, world!")
# Appending
with open('log.txt', 'a') as file:
file.write("New log entry\n")
7. Exception Handling
To handle errors and prevent program crashes.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
except Exception as e:
print(f"An error occurred: {e}")
else:
print("No exceptions")
finally:
print("Always executed")
# Raising exceptions
if x < 0:
raise ValueError("x must be non-negative")
8. Modules and Packages
# Importing entire module
import math
print(math.pi)
# Importing specific functions
from datetime import datetime, timedelta
# Aliasing
import numpy as np
9. Python Cheatsheet for Advanced Concepts
Decorators
Modify the behavior of functions without changing their core logic.
def logging_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__}")
return func(*args, **kwargs)
return wrapper
@logging_decorator
def greet(name):
return f"Hello, {name}!"
Generator Functions
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
# Using generator
for num in fibonacci(10):
print(num)
Lambda Functions
add = lambda x, y: x + y
result = add(5, 3) # result = 8
Context Managers
class FileManager:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
self.file = None
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_value, traceback):
self.file.close()
# Usage
with FileManager('example.txt', 'w') as f:
f.write('Hello, world!')
List Comprehensions
Create lists concisely using list comprehensions.
squares = [x**2 for x in range(10)] # [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
10. Python Cheatsheet for Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm that uses objects and classes to create models based on the real world.
Here are the key OOP terms in Python:
- Function
- Arguments
- Parameters
- Methods
- Attributes
- Encapsulation
- Abstraction
- Composition
- Inheritance
- Aggregation
- Override Methods
- Polymorphism
Function
We talked about this earlier. A function is a part of code that does a particular task. It starts with the word ‘def’ and can be used to run that task.
- A self-contained block of code.
- Can be called independently, without being part of a class or object.
- Typically returns a value.
# Function
def greet(name):
print(f"Hello, {name}!")
# Calling the function
greet("Asad") # Output: Hello, Asad!
Arguments and Parameters
Parameters are the variables listed inside the parentheses of a function definition.
- They receive values when the function is called.
- They serve as placeholders for the real values that will be used.
Arguments are the actual values that you pass to the function when you call it.
# Function with parameters
def greet(name, age): # 'name', 'age' is a parameter
print(f"Hello, {name}! You are {age} years old.")
# Calling
greet("Asad", 25) # 'Asad', 25 is an argument
#Output: Hello, Asad! You are 25 years old.
Methods and Attributes
Methods
Methods are special functions that belong to a class. They work with objects created from that class and are called using a dot, like this: class.method().
class Person:
def greet(self, name): # Method
print(f"Hello, {name}!")
person = Person()
person.greet("Asad") # Output: Hello, Asad!
Attributes
Attributes are pieces of information that belong to a specific object created from a class. They store data about that object.
Here are some important points about attributes:
- Instance attributes: These are tied to a specific object.
- Class attributes: These belong to the class itself and are shared by every object created from that class.
Attributes can be:
- Public: These can be accessed from outside the class.
- Private: These can only be used within the class (usually marked with a _ before the name).
class Vehicle:
def __init__(self, brand, model, year):
self.brand = brand
self.model = model
self.year = year
self.mileage = 0 # instance attribute
num_wheels = 4 # class attribute
# Creating an object
car = Vehicle("Toyota", "Corolla", 2015)
print(car.brand, car.model, car.year) # Output: Toyota Corolla 2015
print(car.num_wheels) # Output: 4
Encapsulation
Encapsulation is when you keep data (like details) and the actions that can be done with that data together in one place called a class. This helps to:
- Keep the inner workings private
- Protect data from outside changes
- Give a safe way to access and change the data
class Person:
def __init__(self, name, age):
self.__name = name # Private attribute
self.__age = age # Private attribute
def get_name(self): # Getter method
return self.__name
def get_age(self): # Getter method
return self.__age
person = Person("Asad", 25)
print(person.get_name()) # Output: Asad
print(person.get_age()) # Output: 25
Abstraction
Abstraction means hiding the complex implementation details and showing only the essential features of the object.
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Abstracted data
def deposit(self, amount):
self.__balance += amount # Abstracted control
def get_balance(self):
return self.__balance # Abstracted interface
Composition
Composition is a way to put together objects or classes to create more complex structures. Simply put, composition is a relationship between objects where one object (the container) holds a collection of other objects. The contained objects depend on the container, which also takes care of how long they last.
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine() # Composition
def start_car(self):
self.engine.start() # Using contained object
my_car = Car()
my_car.start_car() # Output: Engine started
Inheritance
Inheritance is a way for one class (called the subclass) to take on the properties and actions from another class (called the superclass). The subclass gets all the features and functions of the superclass, and it can also add new features or change the ones it inherited.
class Animal:
def sound(self): # Method
print('Generic sound')
class Dog(Animal): # "Inheritance" Dog class inherit from Animal class
def sound(self): # overriding the sound method
print('Bark')
dog = Dog()
dog.sound()
Aggregation
Aggregation means that one object (the container) holds a group of other objects. However, the objects inside the container can live on their own and do not depend on the container to exist.
In simpler terms, aggregation is a less strict version of composition. It allows the objects inside to have their own life and to be used by different containers.
Key differences between Aggregation and Composition:
- Composition: Contained objects cannot exist independently of the container.
- Aggregation: Contained objects can exist independently of the container.
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
class Library:
def __init__(self):
self.books = [] # aggregation
def add_book(self, book):
self.books.append(book)
print(f'{book.title} by {book.author} added to the library')
book1 = Book('The Catcher in the Rye', 'J.D. Salinger')
library = Library()
library.add_book(book1)
book2 = Book('To Kill a Mockingbird', 'Harper Lee')
library.add_book(book2)
Override Methods
In object-oriented programming, an override method is a method in a subclass (a class that is based on another class) that has the same name, return type, and parameter list as a method in its parent class (the class it is based on). The goal of an override method is to provide a specific version for the subclass that is different from the version in the parent class.
When a subclass overrides a method, it means that it is providing its own version of that method. This version will be used instead of the version in the parent class.
class Animal:
def sound(self):
print('Generic sound')
class Dog(Animal):
def sound(self): # overriding the sound method
print('Bark')
dog = Dog()
dog.sound()
Polymorphism
Polymorphism lets you use methods in a similar way, even if they come from different classes and work differently. In Python, polymorphism means you can treat objects from different classes as if they are all from the same parent class.
Types of Polymorphism:
- Method Polymorphism: Methods with the same name but different parameters or behaviors.
- Operator Polymorphism: Operators (+, -, *, /, etc.) can be redefined for custom classes.
- Function Polymorphism: Functions can be defined to work with different types of data.
# Polymorphism -> Present the same interface for different data types.
class Shape:
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self): # Polymorphic method
return (f'area of the circle is {3.14 * self.radius ** 2}')
class Square(Shape):
def __init__(self, side):
self.side = side
def area(self): # Polymorphic method
return (f'area of square is {self.side ** 2}')
shapes = [Circle(5), Square(5)]
for shape in shapes:
print(shape.area())
# Output: area of the circle is 78.5
# area of square is 25
11. Standard Library Highlights
Collections Module
from collections import Counter, defaultdict, namedtuple
# Counting elements
word_counts = Counter(['apple', 'banana', 'apple'])
# Default dictionary
word_count = defaultdict(int)
# Named tuple
Point = namedtuple('Point', ['x', 'y'])
p = Point(10, 20)
Datetime Module
from datetime import datetime, timedelta
now = datetime.now()
future_date = now + timedelta(days=30)
Random Module
import random
# Random selection
random_item = random.choice([1, 2, 3, 4, 5])
random_sample = random.sample(range(100), 5)
- Change Data Capture: Powering Real-time Data Systems
- Deep Learning: A Comprehensive Beginner’s Guide
- Semantic Search in the Age of Generative AI
- Python Projects for Beginners to Building a Portfolio
- Understanding Machine Learning: A Beginner’s Guide to AI’s Most Powerful Tool
Frequently Asked Questions
Q1. Best Python Cheat Sheet PDF.
You can download a printable version of this python cheatsheet in PDF format here
Q2. Advanced python cheat sheet pdf
Download a printable version of your advanced python cheat sheet pdf here
Pingback: CNNs with Self-Supervised Learning: A New Paradigm for Computer Vision - intellitechtribe.com